How to Build a Daily RSS Email Digest
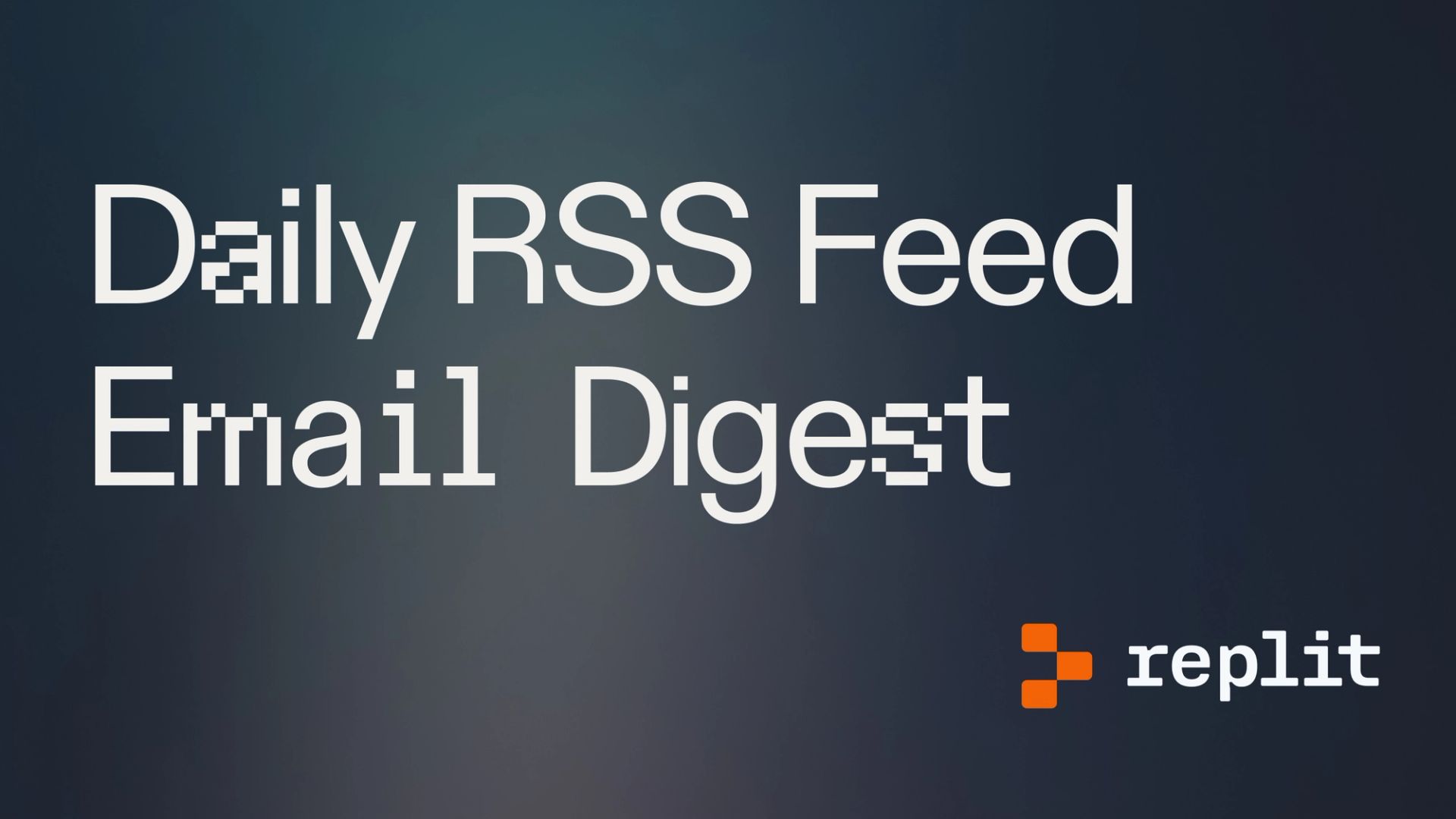
Introduction #
This guide will walk you through creating a Node.js script that fetches RSS feeds, checks for new posts, and sends a digest email using the Resend API. We'll break it down into manageable steps and explain each part of the code.
Getting started #
To get started, fork the template below. The Node.js Repl will come with a few files:
- ReadMe.md - Step-by-step guide similar to this page
- final_code.js - The final project for reference
- index.js - A blank JavaScript file to work from
Creating a Resend API key
First, go to resend.com, and sign up for a free account. Note: You have to sign up for an account with the email you want to send your daily summary too!!
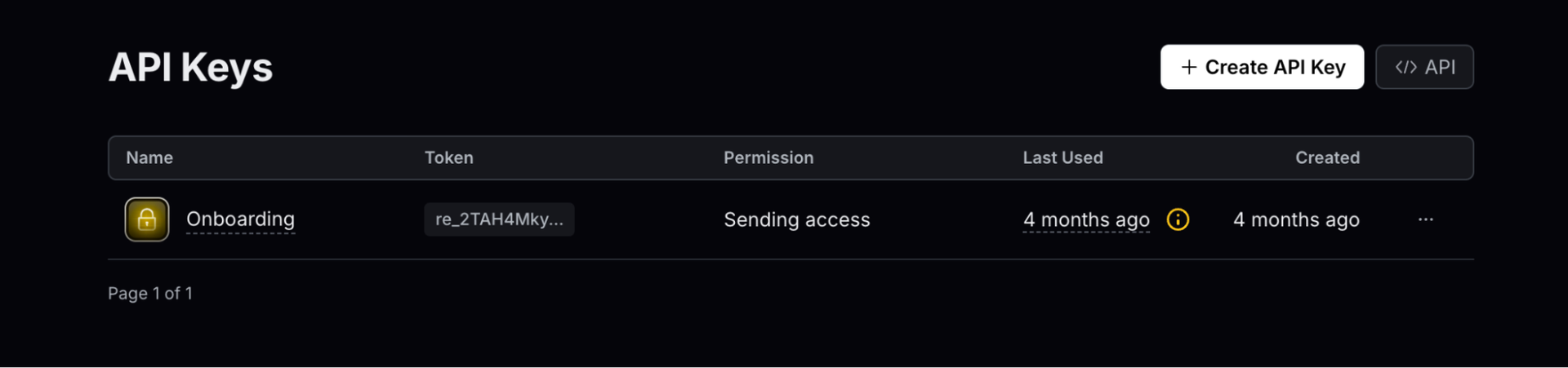
Then go to resend.com/api-keys, and select “Create an API Key.” Give the API key full permissions.
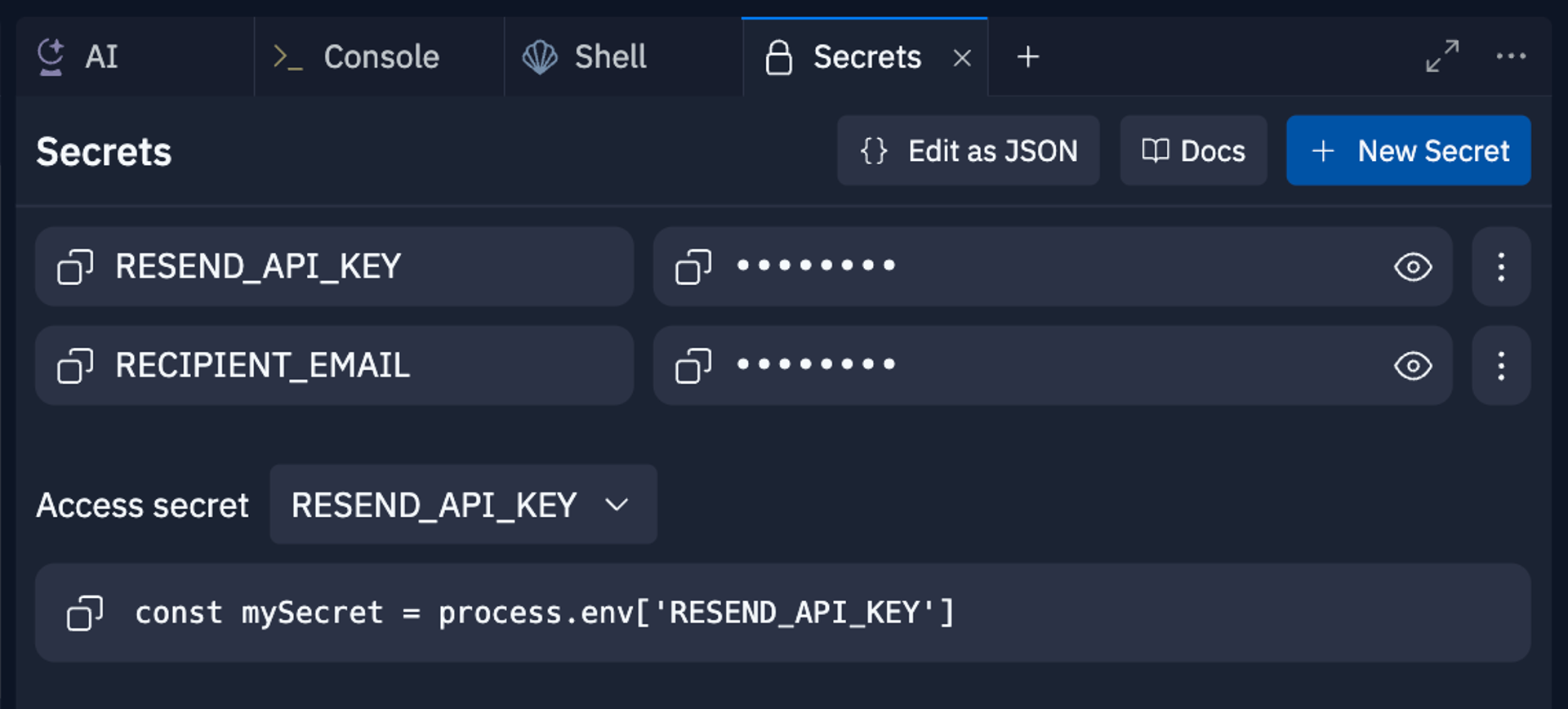
Then paste the API Key into the Secrets tab in your Repl as the secret labeled RESEND_API_KEY. Then add your email to the secret labeled RECIPIENT_EMAIL.
Install the dependencies and secrets
Add the following code to your index.js file.
1
2
3
4
5
6
7
8
const Database = require("@replit/database");
const Parser = require("rss-parser");
const { Resend } = require("resend");
const db = new Database();
const parser = new Parser();
const resend = new Resend(process.env.RESEND_API_KEY);
const recipientEmail = process.env.RECIPIENT_EMAIL;
In this project, we will use an RSS feed parser, Resend, and Replit Database. This code block imports the necessary libraries, and then initializes each one.
Choose your RSS feeds
1
2
3
4
5
6
7
8
9
10
const feeds = [
{
title: "The New York Times", // Feed title
url: "https://rss.nytimes.com/services/xml/rss/nyt/HomePage.xml", // Feed URL
},
{
title: "ABC News",
url: "https://abcnews.go.com/abcnews/usheadlines",
},
];
Next, we will define the RSS feeds we want to monitor. For this example, we will create summaries of new posts to The New York Times and ABC News. You can add, remove, or edit these RSS feeds as much as you would like.
Creating the function to find new posts #
Create the Main Function
Add the following async function to index.js.
1
2
3
(async () => {
// We'll add the main logic here in the next steps
})();
This creates an immediately invoked async function that will contain our main logic.
Fetch the Last Check Time
Inside the main function, add:
1
2
const rawLastCheckTime = (await db.get("lastCheckTime")).value;
const lastCheckTime = rawLastCheckTime ? new Date(rawLastCheckTime) : null;
Each time our function runs, we want to see what new posts the RSS feed has. This section of code checks for the timestamp of the last time the script ran. At the end, we will show you how you can run this daily, weekly, or even every five minutes!
Process RSS Feeds
Still inside the main function, add:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
const digest = [];
for (const feed of feeds) {
const parsedFeed = await parser.parseURL(feed.url);
const latestPosts = parsedFeed.items.slice(0, 5);
let newPosts = [];
if (lastCheckTime) {
newPosts = parsedFeed.items.filter(
(item) => new Date(item.pubDate) > lastCheckTime
);
} else if (latestPosts.length > 0) {
newPosts = latestPosts;
}
if (newPosts.length > 0) {
digest.push({
title: feed.title,
posts: newPosts,
});
}
}
This code processes each RSS feed, checks for new posts since the last check time (or takes the latest five if it's the first run), and adds them to the digest. If you want to take more than five, you can can adjust const latestPosts = parsedFeed.items.slice(0, 5);.
Update the Last Check Time
After processing all feeds, update the last check time:
1
await db.set("lastCheckTime", new Date().toISOString());
This will prevent pulling the same posts over and over.
Send the Email Digest #
Now that we have all of the latest posts, we want to reformat them and send it in a summarized email!
1
2
3
4
if (digest.length === 0) {
console.log("No posts to send");
return;
}
First, we want to check to see if there are posts to send. If there are not, we will avoid sending an email.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
const { data, error } = await resend.emails.send({
from: "My Repl Bot <onboarding@resend.dev>",
to: [recipientEmail],
subject: "RSS Digest",
html: digest
.map((feed) => {
const posts = feed.posts
.map((post) => {
return `<h3><a href="${post.link}">${post.title}</a></h3>
<p>${post.contentSnippet}</p>`;
})
.join("");
return `<h1>${feed.title}</h1>${posts}`;
})
.join(""),
});
console.log(data, error);
If there are posts to send, we will then repackage the posts, and send an email when the job runs. Each email includes the post title, link, and content summary. The posts will be grouped by publisher.
Deploy the application #
Your index.js file should now look the same as final_code.js. This means you’re done! Click run, and you should receive an email digest of the latest posts!
If you want this to run on a set cadence, you will need to deploy it.
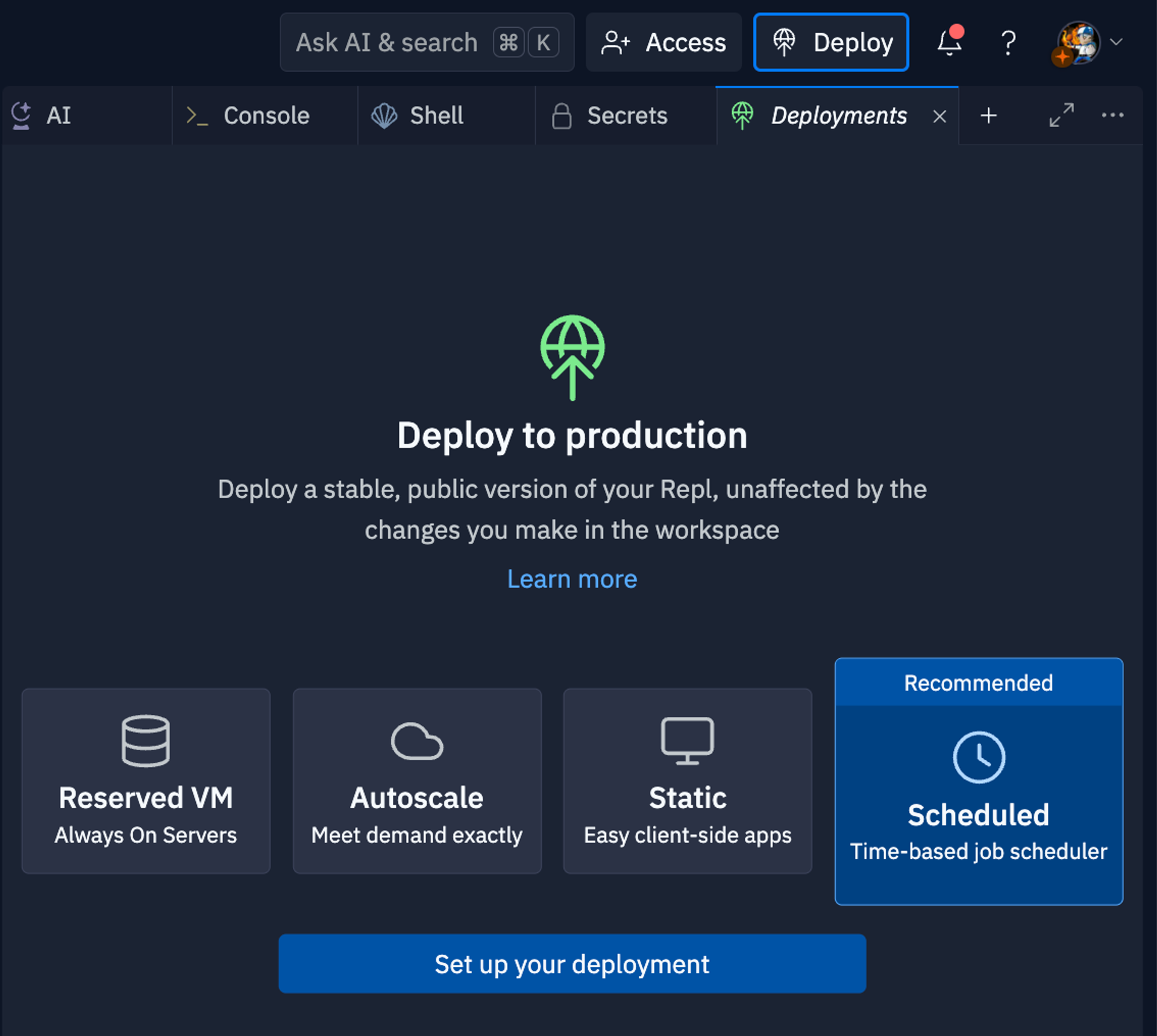
Select “Deploy” in the top-right corner. We want to use a “Scheduled” deployment.
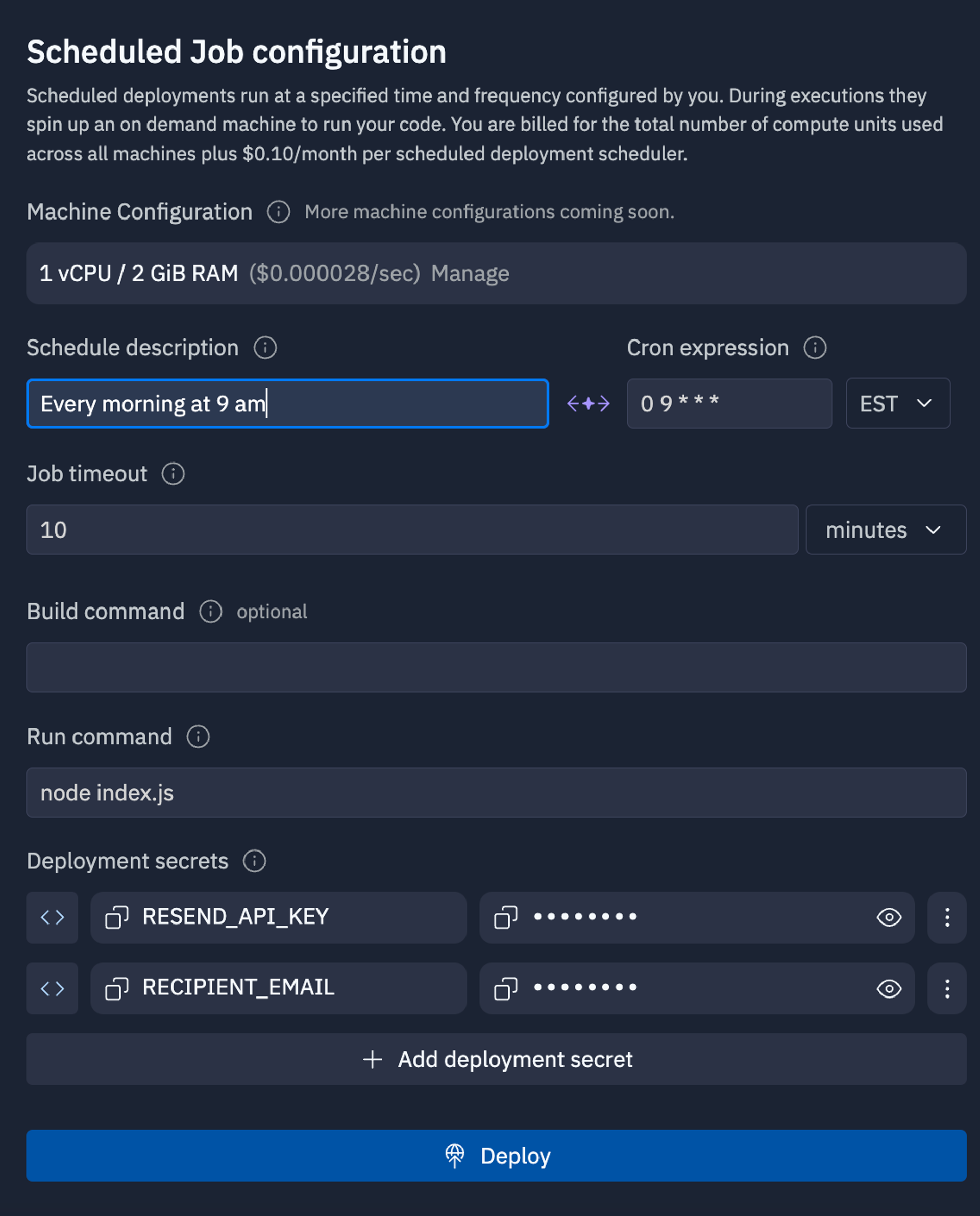
Type in the frequency that you want it to run, and click “Deploy.” Once it successfully deploys, you should be good to go!
What's next #
Keep on building with this idea! Use the built-in Replit AI chat to remix the code and add more features. If you’d like to bring this project and similar templates into your team, set some time here with the Replit team for a quick demo of Replit Teams.
Happy coding!