How to Build a Contact Enrichment App in Slack
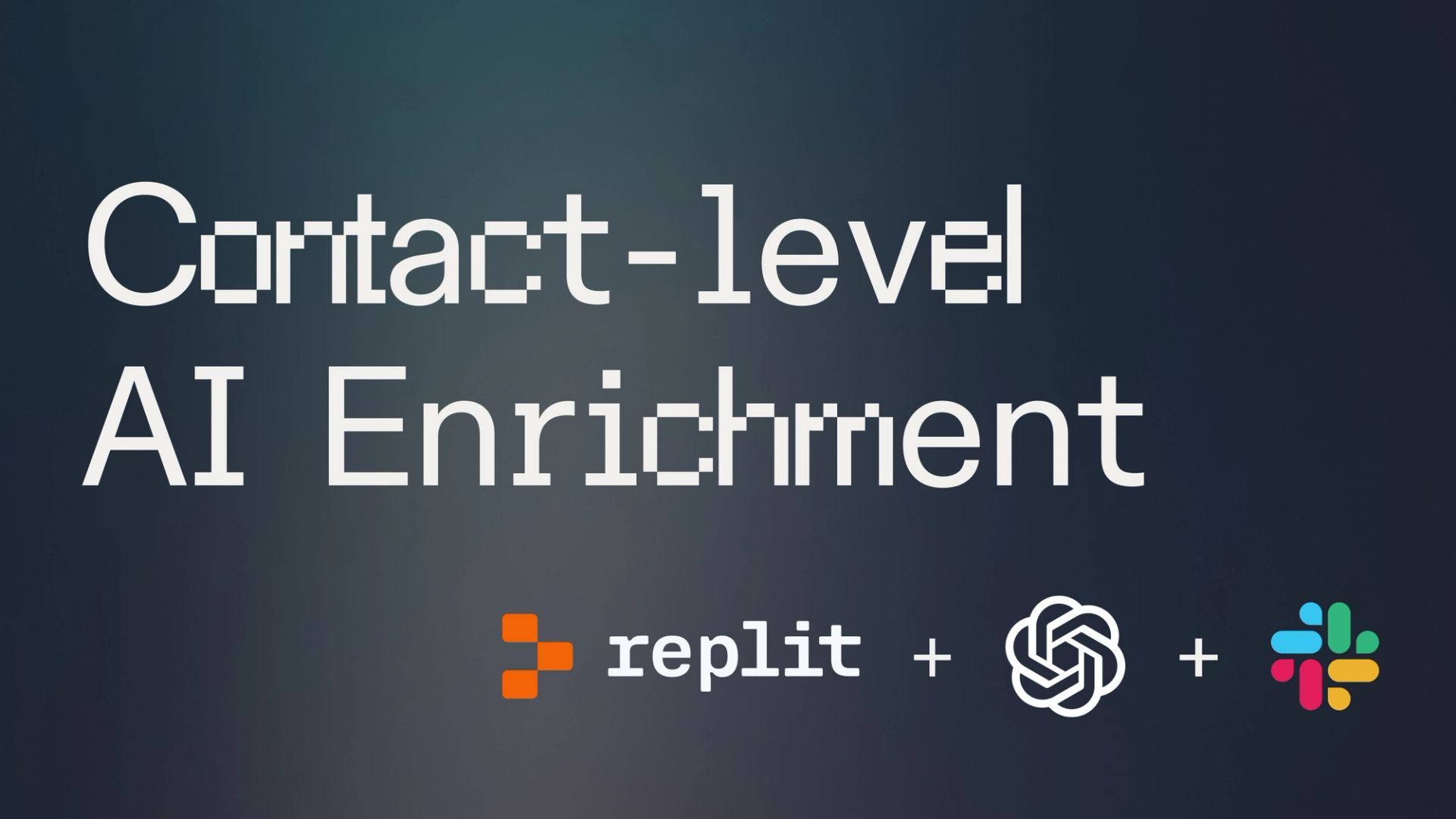
Introduction #
In this guide, you'll learn how to create a Slackbot that monitors user sign-up messages on Slack and uses AI to perform a Google Search automatically on that user to generate insightful summaries of the user's background.
If you're ready to dive in, run, and remix the completed project, you can fork the template here:
Whether you're a developer or a non-technical professional, this guide is designed to make the whole process simple and straightforward using Replit's bundled platform. The entire setup, starting from scratch, can be completed in under 30 minutes. Plus, if you encounter any challenges, you can easily fork the template into Replit and use the built-in AI Chat for assistance.
Getting Started #
Slack App Setup
- Go to the Slack Apps dashboard and create a new app.
- In your app's settings, navigate to the "Basic Information" page.
- Scroll down to the "App-Level Tokens" section and click "Generate Token and Scopes".
- Add the connections write scope for Socket Mode.
- Generate the token and add it to Replit's Secrets tab as SLACK_APP_TOKEN.
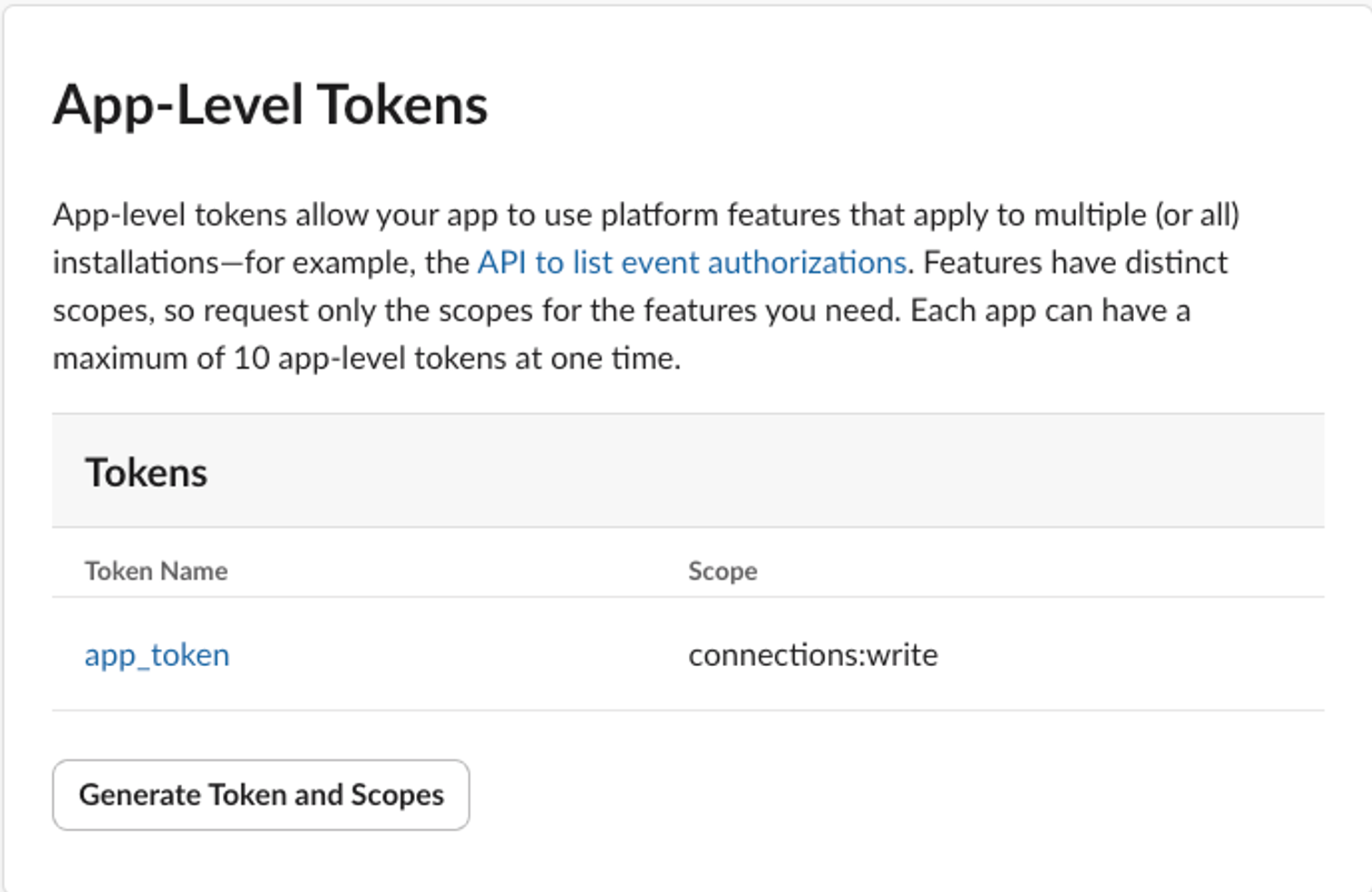
- Navigate to "OAuth & Permissions" and find your "Bot User OAuth Token". Add this to Replit's Secrets as SLACK_BOT_TOKEN.
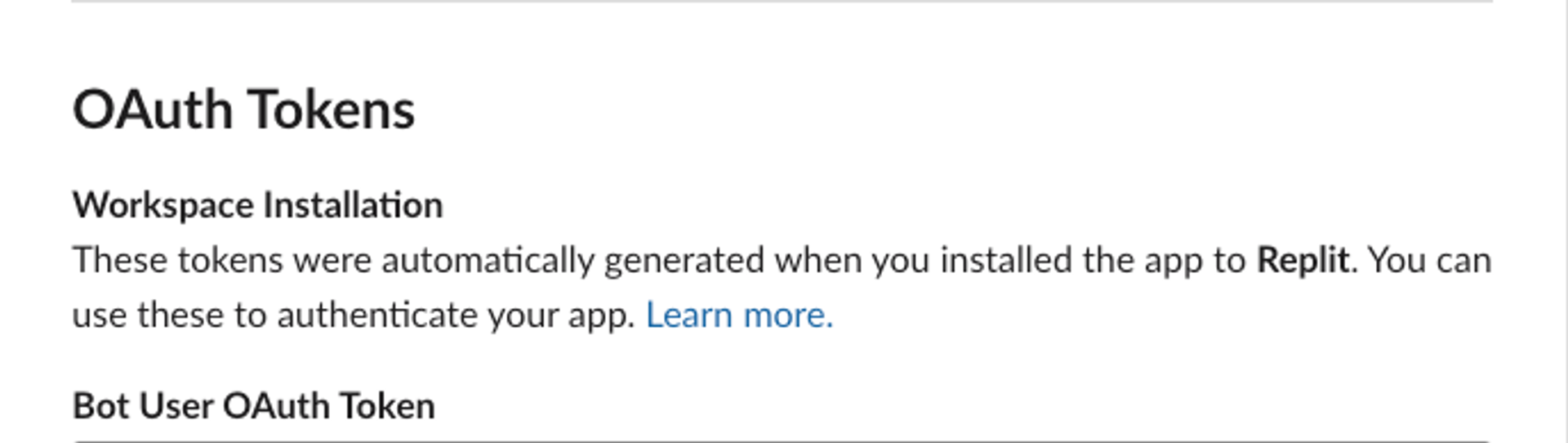
- Set at least the following bot token scopes:
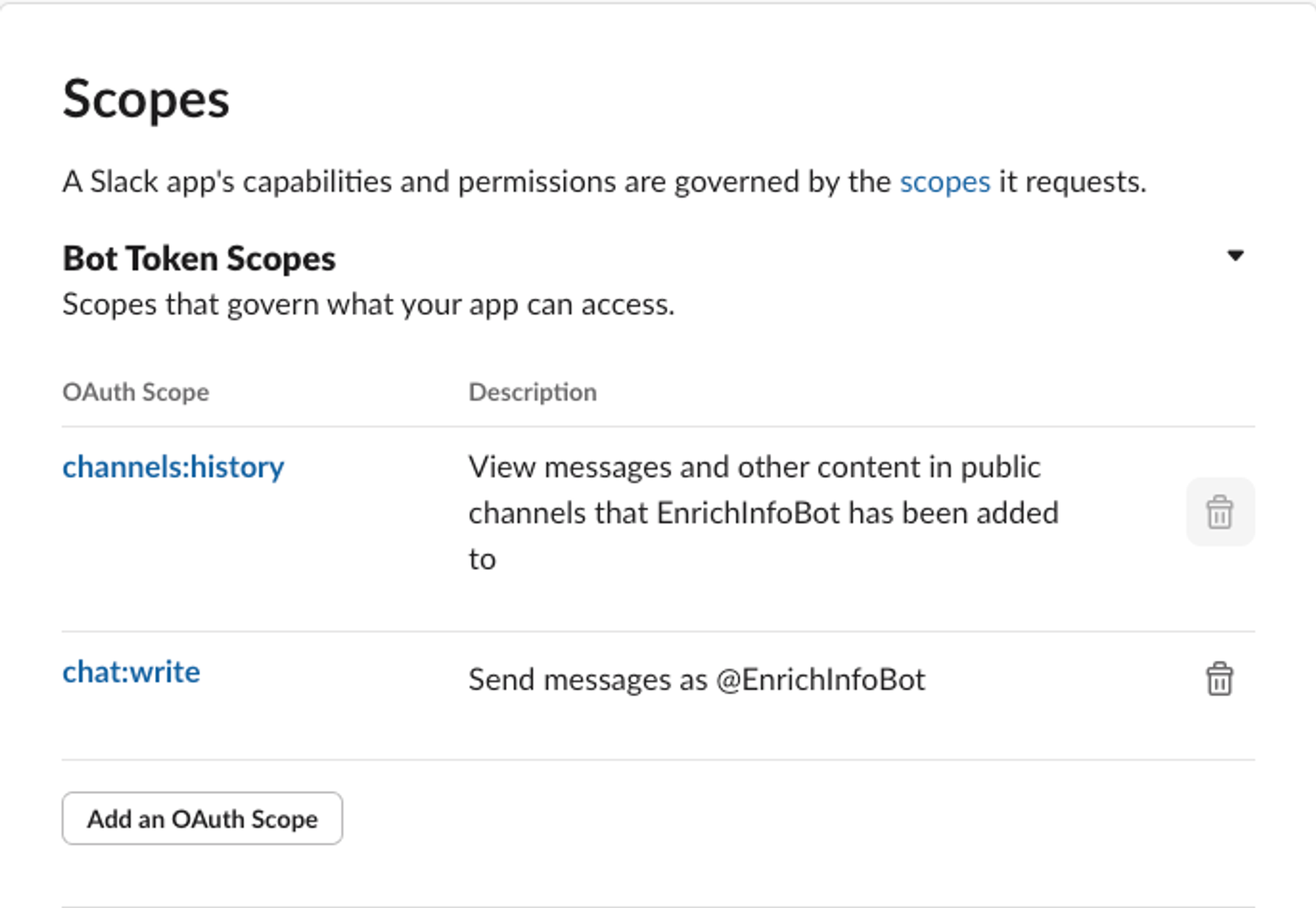
- On your app page, scroll down until you see the ability to "Install App to Workspace." Follow that process.
For more options, it's worth checking out the Slack documentation.
Create Serp API key
Login to Serp API and generate API key. Verify your email and phone number to access the free plan.
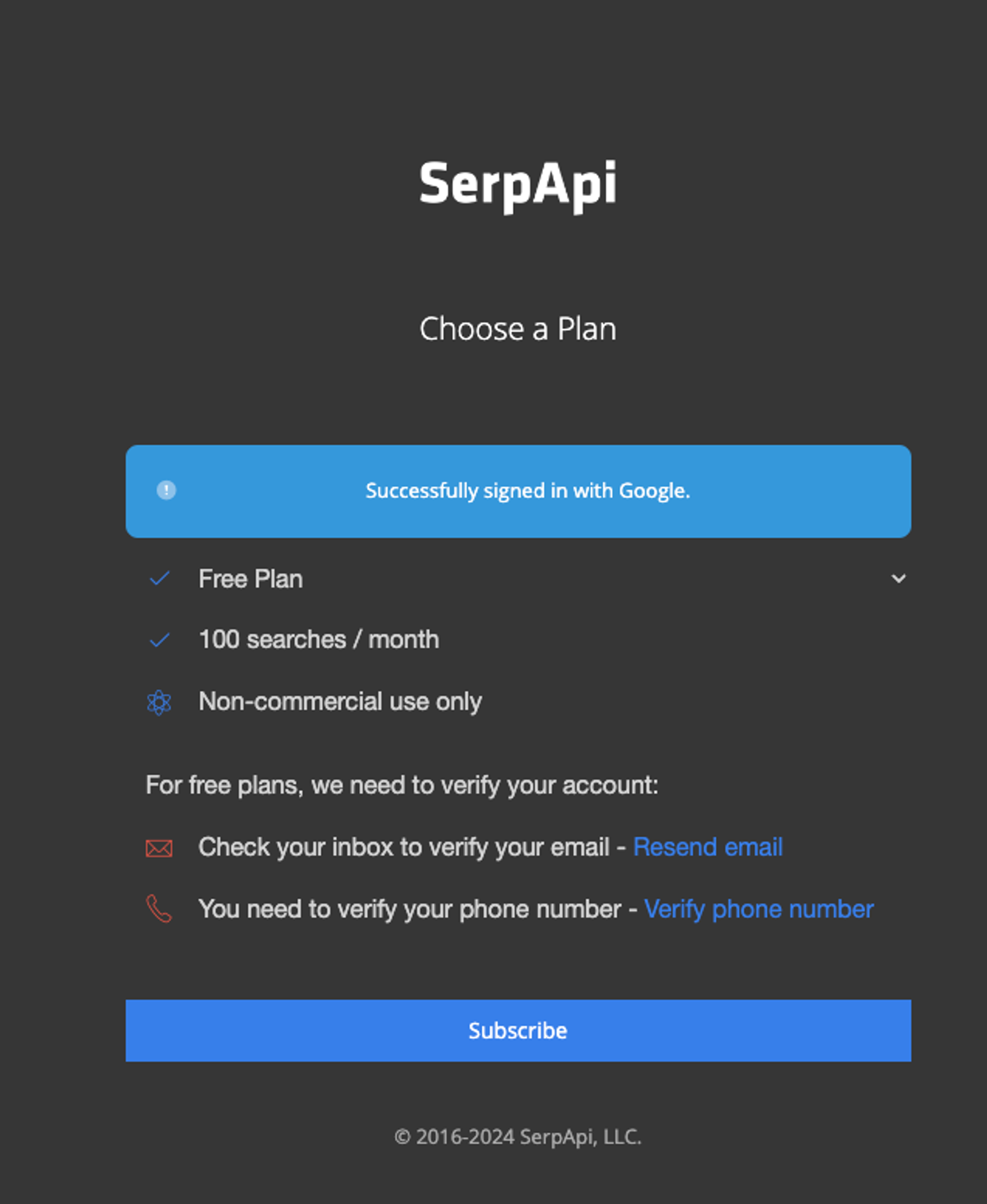
Once you subscribe, you will find your private key on the Serp API dashboard. Add it to Replit's Secrets tab as SERP_API_KEY

Create OpenAI API key #
The first step is to sign up for one OpenAI account. Then proceed to directly the API key page, and create an API Key. Once you have your API Key, go back to your project on Replit and add it to Replit's Secrets tab as OPENAI_API_KEY.
Secrets
Once you complete above steps, you should have secrets added in the secrets pane on Replit.
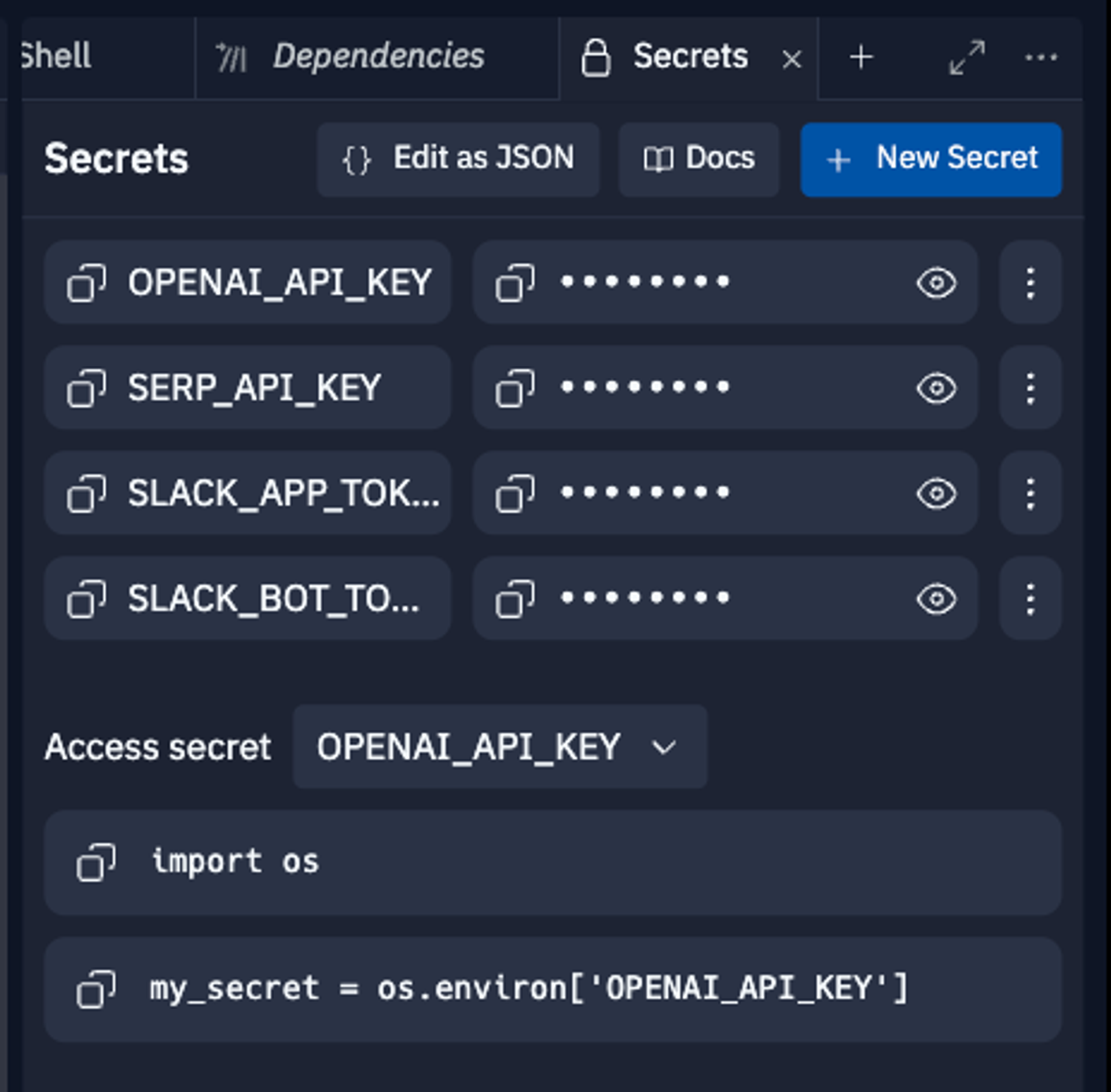
Understanding the Code #
Project uses Slack Bolt framework to listen to the slack messages and send processed response back to the slack channel. Here are the key components:
app.py
This file primarily focuses on initializing the Slack app, setting up event handlers, and defining the logic to process Slack messages
Initializing the Slack App
python
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
SLACK_BOT_TOKEN = os.environ['SLACK_BOT_TOKEN']
SLACK_APP_TOKEN = os.environ['SLACK_APP_TOKEN']
app = App(token=SLACK_BOT_TOKEN)
logging.basicConfig(level=logging.DEBUG)
BOT_ID = app.client.auth_test()
TARGET_CHANNEL_ID = "C07J7TMT53Q"
.
.
.
if __name__ == "__main__":
SocketModeHandler(app, SLACK_APP_TOKEN).start()
- Initializes the Slack app with the SLACK_BOT_TOKEN.
- Sets up logging to DEBUG level for detailed logs.
- Authenticates the bot and retrieves its BOT_ID.
- Defines a TARGET_CHANNEL_ID to specify where the bot should listen for messages.
- Connects the Slack app via Socket Mode using the SLACK_APP_TOKEN to start listening to events and interactions.
Event Handling
python
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
@app.event("message")
def message(event, say, logger):
channel_id = event.get('channel')
user_id = event.get('user')
text = event.get('text')
ts = event.get('ts') # Extract the message timestamp
parent_user_id = event.get('parent_user_id')
# Send message to the target channel and only respond to the top level messages in the channel
if channel_id != TARGET_CHANNEL_ID or parent_user_id is not None:
return
# Ignore messages sent by the bot itself
if user_id is not None and user_id == BOT_ID:
return
email = extract_email(text)
if email is None:
say(channel=channel_id, text="No Admin email found", thread_ts=ts)
# Search user data from serpapi using the email
email_info = search_email_info(email)
linkedin_url = filter_linkedin_url(email_info)
if linkedin_url is None:
say(channel=channel_id, text="No LinkedIn profile found", thread_ts=ts)
# Scrape LinkedIn profile data
user_info = scrape_linkedin(linkedin_url)
# Use OpenAI to generate a summary message
summary_message = generate_sales_message(user_info)
# Post a threaded reply
say(channel=channel_id, text=summary_message, thread_ts=ts)
- Event Listener: The message event listener reads messages from the specified Slack channel.
- Extraction Logic: Extracts relevant information (e.g., admin email) from the message.
- Data Fetching: Uses external modules (scraper, utils) to fetch LinkedIn details.
- Response: Posts a response back to the Slack channel threading the message.
utils.py
This file contains utility functions that help extract and process various types of user information. The main features include extracting admin emails from text, searching for emails using the SerpAPI, and filtering LinkedIn URLs from the search results to enrich user profiles.
You can update the extract_admin_email utility function to extract additional user attributes that could help query more relevant data from the SerpAPI. By expanding the scope of attributes you extract, you can retrieve more detailed and targeted information, which can then be used to further enrich your customer profiles.
While this guide focuses on working with LinkedIn profiles, the utility functions in utils.py can easily be extended to extract additional information, such as GitHub, Facebook, Twitter, and Portfolio URLs, from the user data provided by the Serper API. This makes the utilities versatile and adaptable for enriching user data across multiple platforms, providing a more comprehensive user profile.
scraper.py
This file is responsible for fetching and parsing data from LinkedIn profiles. It includes functions to retrieve HTML content from a LinkedIn URL and extract key details such as the user's name, company, and bio. The current implementation focuses on scraping unauthenticated public profiles, which have limited content available.
You can extend these features to scrape additional information, such as location, employment history, and designations, to build a more detailed user profile. The file provides a foundation for gathering essential LinkedIn data, with the flexibility to adapt as your needs evolve.
summarize.py
This file is designed to generate a short and simple summary of a user's background by interacting with OpenAI's GPT model. It takes in user information, such as name, company, LinkedIn URL, and bio, and passes these details to the GPT model with a prompt designed to create a concise summary. This summary includes key details like the user's role, company, social URLs, and any other relevant information that can be quickly referenced.
How to Extend summarize.py for Different Use Cases
The summarize.py script is highly adaptable. By modifying the role and initial_prompt variables, you can cater to different use cases. Here’s how you can extend it:
- Generating Inbound Sales Messages:
- Role: "You are an AI sales representative specializing in creating personalized inbound sales messages based on user backgrounds. Your messages should highlight key benefits relevant to the user's company and role."
- Initial Prompt: "Based on the following user details, generate a personalized inbound sales message that highlights relevant benefits and encourages further engagement:"
- Creating Personalized Welcome Messages:
- Role: "You are an AI onboarding assistant specializing in crafting personalized welcome messages for new users. Your messages should be warm, welcoming, and include details that show an understanding of the user's background."
- Initial Prompt: "Given the following user details, create a personalized welcome message that makes the user feel valued and understood:"
- Writing Emails for Marketing Campaigns:
- Role: "You are an AI marketing strategist specializing in crafting email content for targeted campaigns. Your emails should be engaging, relevant to the user's background, and designed to encourage specific actions. Also, you are a part of Sales team at Replit therefore your goal should be marketing Replit products."
- Initial Prompt: "Based on the user details provided, write an email that would be part of a marketing campaign, focusing on how our products/services can solve their challenges:"
Running your Bot #
To run your Slackbot:
- Make sure all required secrets are set in the Replit Secrets tab.
- Click the "Run" button in Replit.
- Your bot should now be active in your Slack workspace. You'll see a message "⚡️ Bolt app is running!" in the Console.
To interact with your bot:
- Invite the bot to a Slack channel where you want to use it.
- Consider a case where I have a #signups channel, where I get message in the below format for each user signup
1
2
3
4
New User Created!!
Email: example@gmail.com
Created: 2024-08-25 09:16:18 PDT-0700
Trial: false
- Post above message in the channel.
- Bot should response with the background summary of the user in the thread.
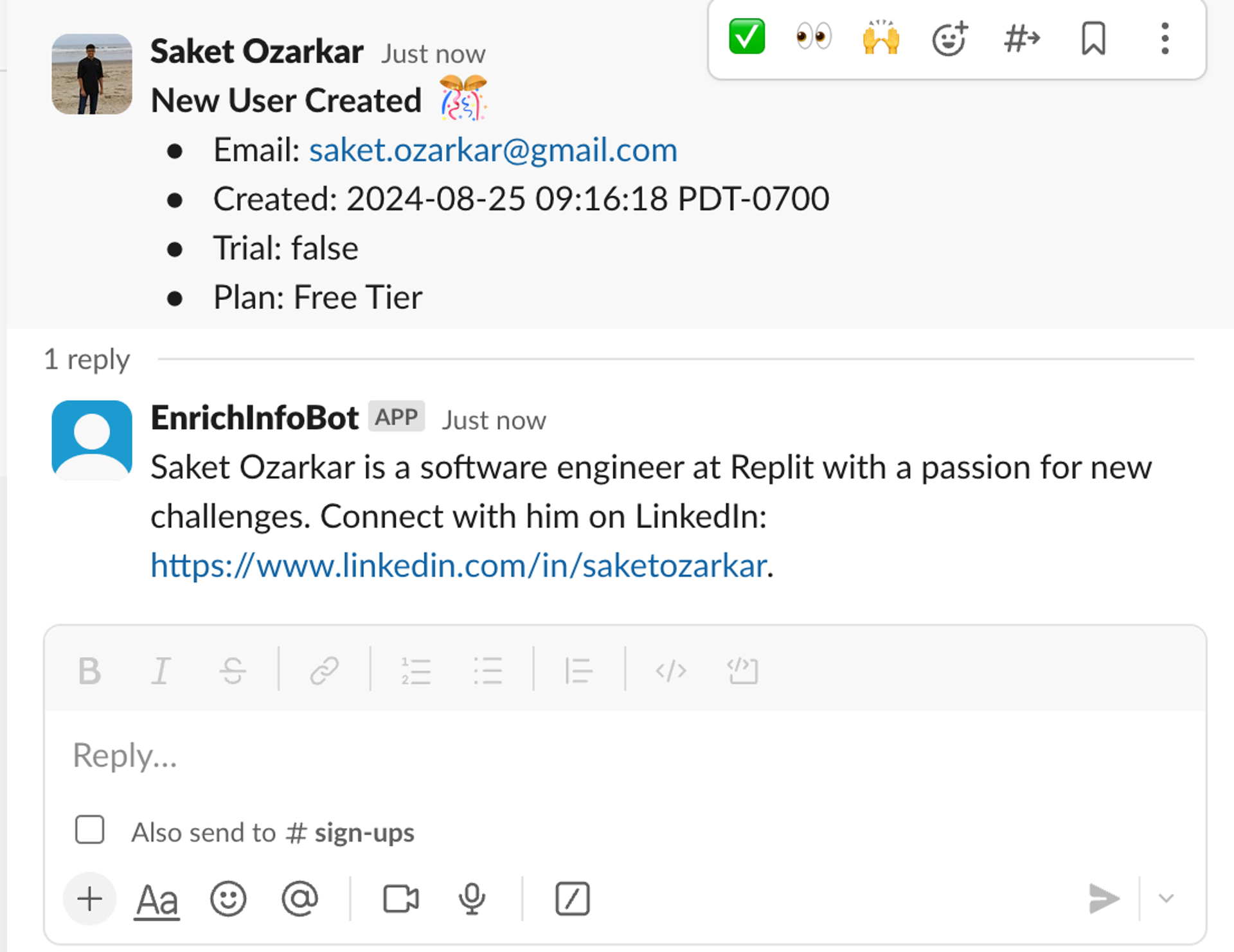
Conclusion #
Congratulations! You’ve just built a powerful application that monitors user sign-up messages on Slack, automatically enriches user data using the Serper API, and generates insightful summaries with the help of LLMs (Large Language Models).
Your app can be extended to handle multiple use cases, such as generating personalized inbound sales messages, automating customer onboarding processes, or creating targeted marketing campaigns.
If you’d like to bring this project and similar templates into your team, set some time here with the Replit team for a quick demo of Replit Teams.
Happy coding!